# Setting up the new R environment, starting fresh, click run!
rm(list=ls())
library(tidyverse)
── Attaching packages ─────────────────────────────────────── tidyverse 1.3.2 ──
✔ ggplot2 3.4.0 ✔ purrr 0.3.4
✔ tibble 3.1.6 ✔ dplyr 1.0.10
✔ tidyr 1.2.0 ✔ stringr 1.4.0
✔ readr 2.1.1 ✔ forcats 0.5.1
── Conflicts ────────────────────────────────────────── tidyverse_conflicts() ──
✖ dplyr::filter() masks stats::filter()
✖ dplyr::lag() masks stats::lag()
# Horizontal Bar Plot
head(mtcars)
mpg cyl disp hp drat wt qsec vs am gear carb
Mazda RX4 21.0 6 160 110 3.90 2.620 16.46 0 1 4 4
Mazda RX4 Wag 21.0 6 160 110 3.90 2.875 17.02 0 1 4 4
Datsun 710 22.8 4 108 93 3.85 2.320 18.61 1 1 4 1
Hornet 4 Drive 21.4 6 258 110 3.08 3.215 19.44 1 0 3 1
Hornet Sportabout 18.7 8 360 175 3.15 3.440 17.02 0 0 3 2
Valiant 18.1 6 225 105 2.76 3.460 20.22 1 0 3 1
counts <- table(mtcars$gear)
barplot(counts, main="Car Distribution", horiz=TRUE, names.arg=c("3 Gears", "4 Gears", "5 Gears"))
# Column Chart
summary_data <- tapply(mtcars$hp, list(cylinders = mtcars$cyl, transmission = mtcars$am), FUN = mean, na.rm = TRUE)
par(mar = c(5, 5, 4, 10))
barplot(summary_data, xlab = "Transmission type",
main = "Horsepower Mean",
col = rainbow(3),
beside = TRUE,
legend.text = rownames(summary_data),
args.legend = list(title = "Cylinders", x = "topright",
inset = c(-0.20, 0)))
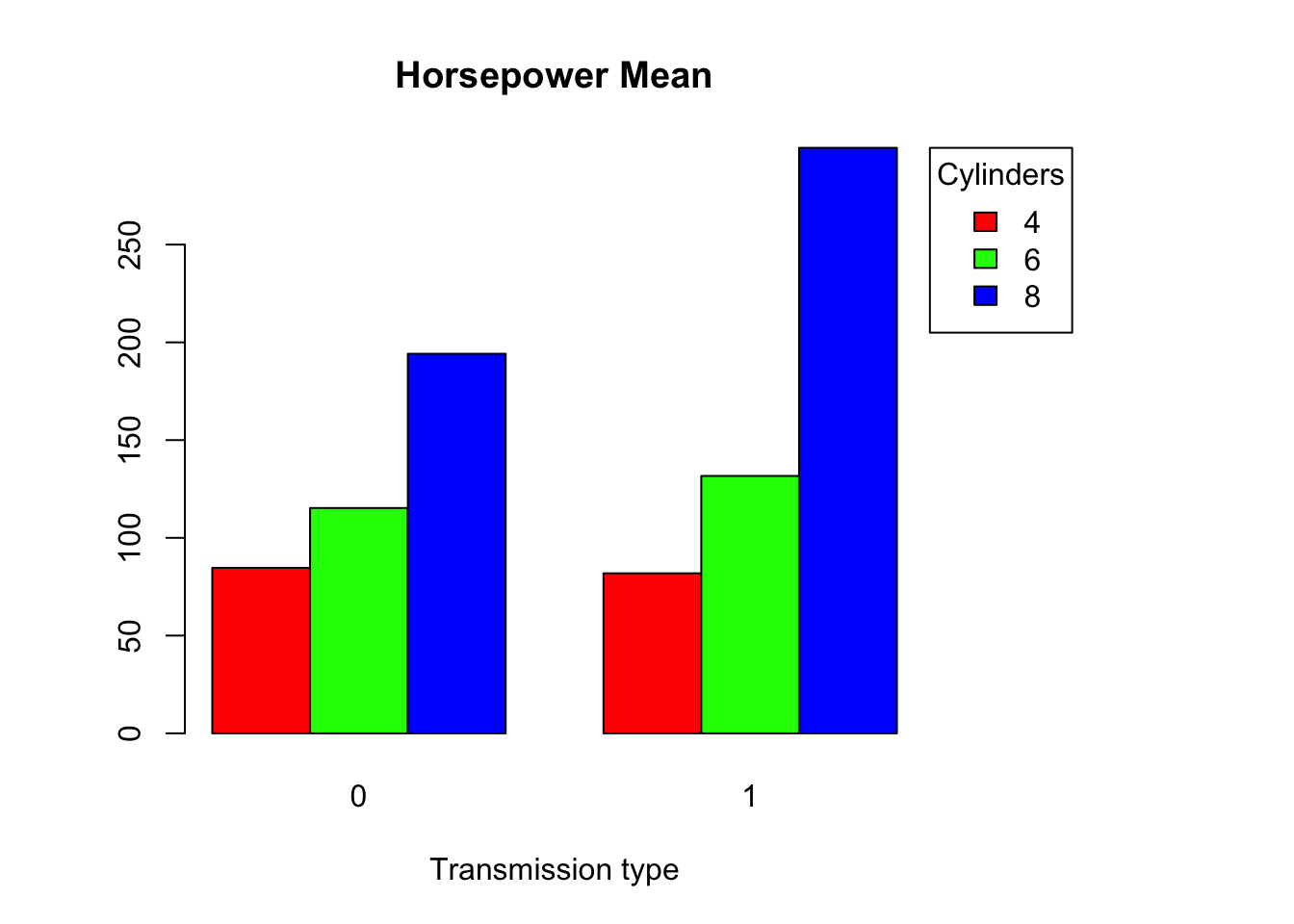
# Radar Chart
library(fmsb)
exam_scores <- data.frame(
row.names = c("Student.1", "Student.2", "Student.3"),
Biology = c(7.9, 3.9, 9.4),
Physics = c(10, 20, 0),
Maths = c(3.7, 11.5, 2.5),
Sport = c(8.7, 20, 4),
English = c(7.9, 7.2, 12.4),
Geography = c(6.4, 10.5, 6.5),
Art = c(2.4, 0.2, 9.8),
Programming = c(0, 0, 20),
Music = c(20, 20, 20)
)
# Define the variable ranges: maximum and minimum
max_min <- data.frame(
Biology = c(20, 0), Physics = c(20, 0), Maths = c(20, 0),
Sport = c(20, 0), English = c(20, 0), Geography = c(20, 0),
Art = c(20, 0), Programming = c(20, 0), Music = c(20, 0)
)
rownames(max_min) <- c("Max", "Min")
# Bind the variable ranges to the data
df2 <- rbind(max_min, exam_scores)
df2
Biology Physics Maths Sport English Geography Art Programming Music
Max 20.0 20 20.0 20.0 20.0 20.0 20.0 20 20
Min 0.0 0 0.0 0.0 0.0 0.0 0.0 0 0
Student.1 7.9 10 3.7 8.7 7.9 6.4 2.4 0 20
Student.2 3.9 20 11.5 20.0 7.2 10.5 0.2 0 20
Student.3 9.4 0 2.5 4.0 12.4 6.5 9.8 20 20
# Generating radar chart
student1_data <- df2[c("Max", "Min", "Student.1"), ]
radarchart(student1_data)